Características
Microcontrolador: ESP-8266 32-bit (Procesador Tensilica Xtensa LX106 de 1 núcleo)
Frecuencia de reloj: 80 MHz
Voltaje de operación: 3,3V
Voltaje de entrada: entre 7V y 12V
Memoria:
- SRAM (memoria volatil): 64 KB
- FLASH (memoria no volatil): 4 MB
Pins I/O digitales: 16
Pins I/O analógicos: 1
Interface UART: 1
Interface SPI: 1
Interface I2C: 1
WiFi: 802.11 b/g/n. Autenticación WEB/WPA/WPA2
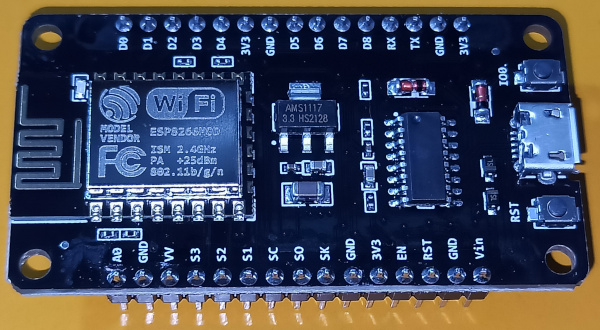
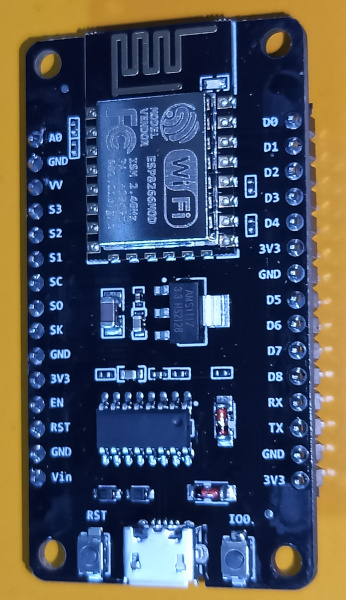
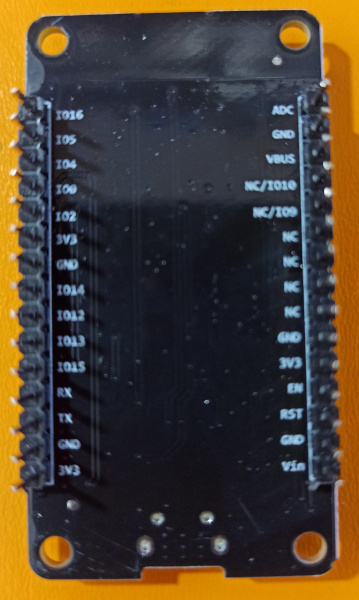
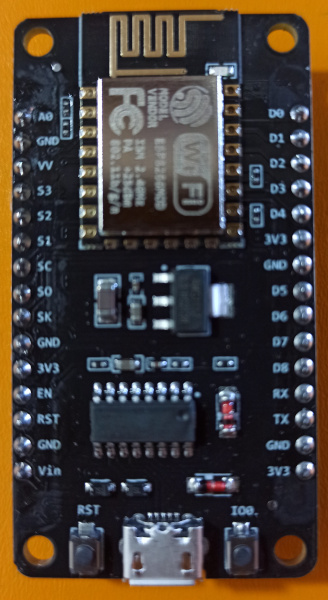
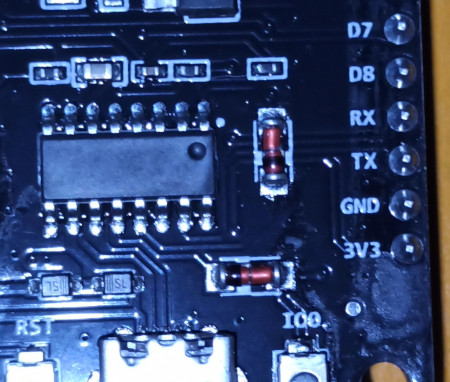
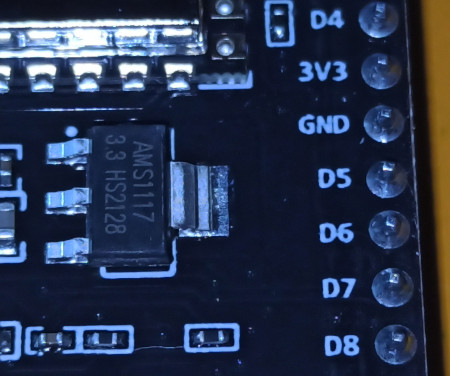
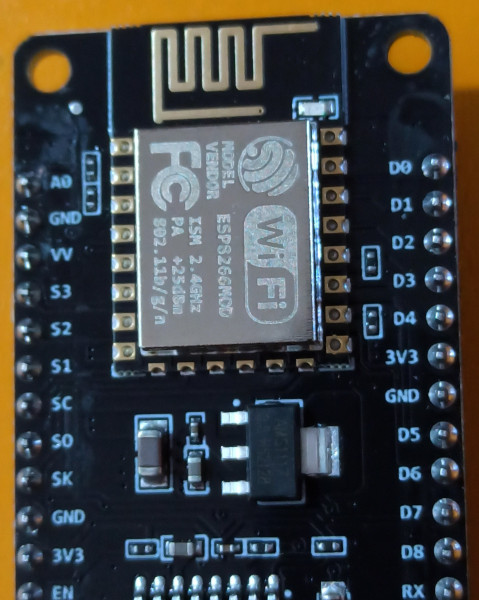
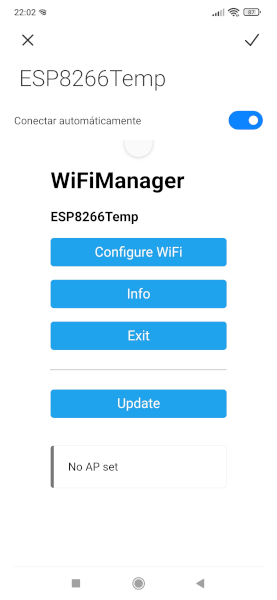
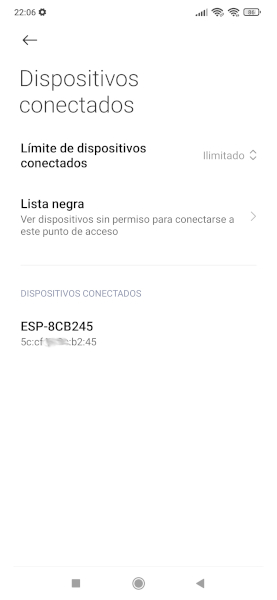
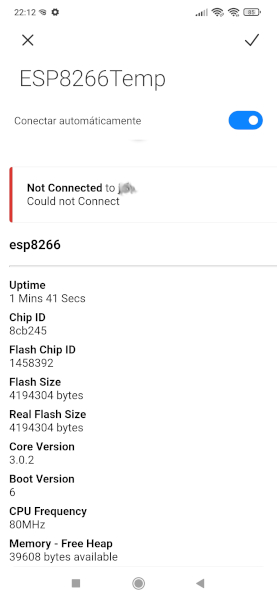
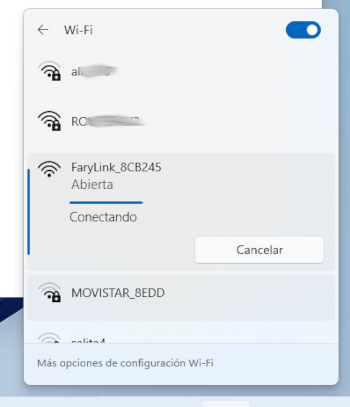
Programas para hacer pruebas...
http://seta43.blogspot.com/2020/01/esp8266-webserver-encender-led-desde_18.html
Pruebas NodeMCU v3 funcionando como servodpr web que transmite el valor de un potenciómetro.
Montaje realizado siguiendo las indicaciones y programa de la página web: https://www.esploradores.com/practica-14-websockets-2/
Pruebas NodeMCU v3 funcionando como servodpr web que transmite el valor de un potenciómetro de 10 KOhms. Conexión wifi tipo "Access Point".
Ver vídeo del montaje: "potenciometro.mp4" (tamaño: 33,5 MB)
Algunos programas para probar la NodeMCUv3:
void setup() { pinMode(D4, OUTPUT); } void loop() { digitalWrite(D4, HIGH); delay(1000); digitalWrite(D4, LOW); delay(1000); }
#include "ESP8266WiFi.h" void setup() { Serial.begin(115200); delay(1500); WiFi.mode(WIFI_STA); WiFi.disconnect(); delay(100); Serial.println("Configuracion completa"); } void loop() { Serial.println("Buscando WIFIs"); // WiFi.scanNetworks will return the number of networks found int n = WiFi.scanNetworks(); Serial.println("Listo"); if (n == 0) Serial.println("No encuentro redes disponibles"); else { Serial.print(n); Serial.println(" networks found"); for (int i = 0; i < n; ++i) { // Print SSID y RSSI para cada una Serial.print(i + 1); Serial.print(": "); Serial.print(WiFi.SSID(i)); Serial.print(" ("); Serial.print(WiFi.RSSI(i)); Serial.print(")"); Serial.println((WiFi.encryptionType(i) == ENC_TYPE_NONE)?" ":"*"); delay(10); } } Serial.println(""); // Wait a bit before scanning again delay(50000); }
/* This sketch demonstrates how to set up a simple HTTP-like server. The server will set a GPIO pin depending on the request http://server_ip/gpio/0 will set the GPIO2 low, http://server_ip/gpio/1 will set the GPIO2 high server_ip is the IP address of the ESP8266 module, will be printed to Serial when the module is connected. */ #include <ESP8266WiFi.h> #ifndef STASSID #define STASSID "NOMBRE_DEL_ROUTER" #define STAPSK "CLAVE_DEL_ROUTER" #endif const char* ssid = STASSID; const char* password = STAPSK; // Create an instance of the server // specify the port to listen on as an argument WiFiServer server(80); void setup() { Serial.begin(115200); // prepare LED pinMode(LED_BUILTIN, OUTPUT); digitalWrite(LED_BUILTIN, 0); // Connect to WiFi network Serial.println(); Serial.println(); Serial.print(F("Connecting to ")); Serial.println(ssid); WiFi.mode(WIFI_STA); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print(F(".")); } Serial.println(); Serial.println(F("WiFi connected")); // Start the server server.begin(); Serial.println(F("Server started")); // Print the IP address Serial.println(WiFi.localIP()); } void loop() { // Check if a client has connected WiFiClient client = server.available(); if (!client) { return; } Serial.println(F("new client")); client.setTimeout(5000); // default is 1000 // Read the first line of the request String req = client.readStringUntil('\r'); Serial.println(F("request: ")); Serial.println(req); // Match the request int val; if (req.indexOf(F("/gpio/0")) != -1) { val = 0; } else if (req.indexOf(F("/gpio/1")) != -1) { val = 1; } else { Serial.println(F("invalid request")); val = digitalRead(LED_BUILTIN); } // Set LED according to the request digitalWrite(LED_BUILTIN, val); // read/ignore the rest of the request // do not client.flush(): it is for output only, see below while (client.available()) { // byte by byte is not very efficient client.read(); } // Send the response to the client // it is OK for multiple small client.print/write, // because nagle algorithm will group them into one single packet client.print(F("HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<!DOCTYPE HTML>\r\n<html>\r\nGPIO is now ")); client.print((val) ? F("high") : F("low")); client.print(F("<br><br>Click <a href='http://")); client.print(WiFi.localIP()); client.print(F("/gpio/1'>here</a> to switch LED GPIO on, or <a href='http://")); client.print(WiFi.localIP()); client.print(F("/gpio/0'>here</a> to switch LED GPIO off.</html>")); // The client will actually be *flushed* then disconnected // when the function returns and 'client' object is destroyed (out-of-scope) // flush = ensure written data are received by the other side Serial.println(F("Disconnecting from client")); }
HelloTcpIp.ino
#define ESP8266WIFIMESH_DISABLE_COMPATIBILITY // Excludes redundant compatibility code. TODO: Should be used for new code until the compatibility code is removed with release 3.0.0 of the Arduino core. #include <ESP8266WiFi.h> #include <TcpIpMeshBackend.h> #include <EspnowMeshBackend.h> #include <TypeConversionFunctions.h> #include <assert.h> namespace TypeCast = MeshTypeConversionFunctions; /** NOTE: Although we could define the strings below as normal String variables, here we are using PROGMEM combined with the FPSTR() macro (and also just the F() macro further down in the file). The reason is that this approach will place the strings in flash memory which will help save RAM during program execution. Reading strings from flash will be slower than reading them from RAM, but this will be a negligible difference when printing them to Serial. More on F(), FPSTR() and PROGMEM: https://github.com/esp8266/Arduino/issues/1143 https://arduino-esp8266.readthedocs.io/en/latest/PROGMEM.html */ constexpr char exampleMeshName[] PROGMEM = "MeshNode_"; constexpr char exampleWiFiPassword[] PROGMEM = "CLAVE_DEL_ROUTER"; // Note: " is an illegal character. The password has to be min 8 and max 64 characters long, otherwise an AP which uses it will not be found during scans. unsigned int requestNumber = 0; unsigned int responseNumber = 0; String manageRequest(const String &request, MeshBackendBase &meshInstance); TransmissionStatusType manageResponse(const String &response, MeshBackendBase &meshInstance); void networkFilter(int numberOfNetworks, MeshBackendBase &meshInstance); /* Create the mesh node object */ TcpIpMeshBackend tcpIpNode = TcpIpMeshBackend(manageRequest, manageResponse, networkFilter, FPSTR(exampleWiFiPassword), FPSTR(exampleMeshName), TypeCast::uint64ToString(ESP.getChipId()), true); /** Callback for when other nodes send you a request @param request The request string received from another node in the mesh @param meshInstance The MeshBackendBase instance that called the function. @return The string to send back to the other node. For ESP-NOW, return an empty string ("") if no response should be sent. */ String manageRequest(const String &request, MeshBackendBase &meshInstance) { // To get the actual class of the polymorphic meshInstance, do as follows (meshBackendCast replaces dynamic_cast since RTTI is disabled) if (EspnowMeshBackend *espnowInstance = TypeCast::meshBackendCast<EspnowMeshBackend *>(&meshInstance)) { String transmissionEncrypted = espnowInstance->receivedEncryptedTransmission() ? F(", Encrypted transmission") : F(", Unencrypted transmission"); Serial.print(String(F("ESP-NOW (")) + espnowInstance->getSenderMac() + transmissionEncrypted + F("): ")); } else if (TcpIpMeshBackend *tcpIpInstance = TypeCast::meshBackendCast<TcpIpMeshBackend *>(&meshInstance)) { (void)tcpIpInstance; // This is useful to remove a "unused parameter" compiler warning. Does nothing else. Serial.print(F("TCP/IP: ")); } else { Serial.print(F("UNKNOWN!: ")); } /* Print out received message */ // Only show first 100 characters because printing a large String takes a lot of time, which is a bad thing for a callback function. // If you need to print the whole String it is better to store it and print it in the loop() later. // Note that request.substring will not work as expected if the String contains null values as data. Serial.print(F("Request received: ")); Serial.println(request.substring(0, 100)); /* return a string to send back */ return (String(F("Hello world response #")) + String(responseNumber++) + F(" from ") + meshInstance.getMeshName() + meshInstance.getNodeID() + F(" with AP MAC ") + WiFi.softAPmacAddress() + String('.')); } /** Callback for when you get a response from other nodes @param response The response string received from another node in the mesh @param meshInstance The MeshBackendBase instance that called the function. @return The status code resulting from the response, as an int */ TransmissionStatusType manageResponse(const String &response, MeshBackendBase &meshInstance) { TransmissionStatusType statusCode = TransmissionStatusType::TRANSMISSION_COMPLETE; // To get the actual class of the polymorphic meshInstance, do as follows (meshBackendCast replaces dynamic_cast since RTTI is disabled) if (EspnowMeshBackend *espnowInstance = TypeCast::meshBackendCast<EspnowMeshBackend *>(&meshInstance)) { String transmissionEncrypted = espnowInstance->receivedEncryptedTransmission() ? F(", Encrypted transmission") : F(", Unencrypted transmission"); Serial.print(String(F("ESP-NOW (")) + espnowInstance->getSenderMac() + transmissionEncrypted + F("): ")); } else if (TcpIpMeshBackend *tcpIpInstance = TypeCast::meshBackendCast<TcpIpMeshBackend *>(&meshInstance)) { Serial.print(F("TCP/IP: ")); // Getting the sent message like this will work as long as ONLY(!) TCP/IP is used. // With TCP/IP the response will follow immediately after the request, so the stored message will not have changed. // With ESP-NOW there is no guarantee when or if a response will show up, it can happen before or after the stored message is changed. // So for ESP-NOW, adding unique identifiers in the response and request is required to associate a response with a request. Serial.print(F("Request sent: ")); Serial.println(tcpIpInstance->getCurrentMessage().substring(0, 100)); } else { Serial.print(F("UNKNOWN!: ")); } /* Print out received message */ // Only show first 100 characters because printing a large String takes a lot of time, which is a bad thing for a callback function. // If you need to print the whole String it is better to store it and print it in the loop() later. // Note that response.substring will not work as expected if the String contains null values as data. Serial.print(F("Response received: ")); Serial.println(response.substring(0, 100)); return statusCode; } /** Callback used to decide which networks to connect to once a WiFi scan has been completed. @param numberOfNetworks The number of networks found in the WiFi scan. @param meshInstance The MeshBackendBase instance that called the function. */ void networkFilter(int numberOfNetworks, MeshBackendBase &meshInstance) { // Note that the network index of a given node may change whenever a new scan is done. for (int networkIndex = 0; networkIndex < numberOfNetworks; ++networkIndex) { String currentSSID = WiFi.SSID(networkIndex); int meshNameIndex = currentSSID.indexOf(meshInstance.getMeshName()); /* Connect to any _suitable_ APs which contain meshInstance.getMeshName() */ if (meshNameIndex >= 0) { uint64_t targetNodeID = TypeCast::stringToUint64(currentSSID.substring(meshNameIndex + meshInstance.getMeshName().length())); if (targetNodeID < TypeCast::stringToUint64(meshInstance.getNodeID())) { if (EspnowMeshBackend *espnowInstance = TypeCast::meshBackendCast<EspnowMeshBackend *>(&meshInstance)) { espnowInstance->connectionQueue().emplace_back(networkIndex); } else if (TcpIpMeshBackend *tcpIpInstance = TypeCast::meshBackendCast<TcpIpMeshBackend *>(&meshInstance)) { tcpIpInstance->connectionQueue().emplace_back(networkIndex); } else { Serial.println(F("Invalid mesh backend!")); } } } } } /** Once passed to the setTransmissionOutcomesUpdateHook method of the TCP/IP backend, this function will be called after each update of the latestTransmissionOutcomes vector during attemptTransmission. (which happens after each individual transmission has finished) Example use cases is modifying getMessage() between transmissions, or aborting attemptTransmission before all nodes in the connectionQueue have been contacted. @param meshInstance The MeshBackendBase instance that called the function. @return True if attemptTransmission should continue with the next entry in the connectionQueue. False if attemptTransmission should stop. */ bool exampleTransmissionOutcomesUpdateHook(MeshBackendBase &meshInstance) { // The default hook only returns true and does nothing else. if (TcpIpMeshBackend *tcpIpInstance = TypeCast::meshBackendCast<TcpIpMeshBackend *>(&meshInstance)) { if (tcpIpInstance->latestTransmissionOutcomes().back().transmissionStatus() == TransmissionStatusType::TRANSMISSION_COMPLETE) { // Our last request got a response, so time to create a new request. meshInstance.setMessage(String(F("Hello world request #")) + String(++requestNumber) + F(" from ") + meshInstance.getMeshName() + meshInstance.getNodeID() + String('.')); } } else { Serial.println(F("Invalid mesh backend!")); } return true; } void setup() { // Prevents the flash memory from being worn out, see: https://github.com/esp8266/Arduino/issues/1054 . // This will however delay node WiFi start-up by about 700 ms. The delay is 900 ms if we otherwise would have stored the WiFi network we want to connect to. WiFi.persistent(false); Serial.begin(115200); Serial.println(); Serial.println(); Serial.println(F("Note that this library can use static IP:s for the nodes to speed up connection times.\n" "Use the setStaticIP method as shown in this example to enable this.\n" "Ensure that nodes connecting to the same AP have distinct static IP:s.\n" "Also, remember to change the default mesh network password!\n\n")); Serial.println(F("Setting up mesh node...")); /* Initialise the mesh node */ tcpIpNode.begin(); tcpIpNode.activateAP(); // Each AP requires a separate server port. tcpIpNode.setStaticIP(IPAddress(192, 168, 4, 22)); // Activate static IP mode to speed up connection times. // Storing our message in the TcpIpMeshBackend instance is not required, but can be useful for organizing code, especially when using many TcpIpMeshBackend instances. // Note that calling the multi-recipient tcpIpNode.attemptTransmission will replace the stored message with whatever message is transmitted. tcpIpNode.setMessage(String(F("Hello world request #")) + String(requestNumber) + F(" from ") + tcpIpNode.getMeshName() + tcpIpNode.getNodeID() + String('.')); tcpIpNode.setTransmissionOutcomesUpdateHook(exampleTransmissionOutcomesUpdateHook); } int32_t timeOfLastScan = -10000; void loop() { if (millis() - timeOfLastScan > 3000 // Give other nodes some time to connect between data transfers. || (WiFi.status() != WL_CONNECTED && millis() - timeOfLastScan > 2000)) { // Scan for networks with two second intervals when not already connected. // attemptTransmission(message, scan, scanAllWiFiChannels, concludingDisconnect, initialDisconnect = false) tcpIpNode.attemptTransmission(tcpIpNode.getMessage(), true, false, false); timeOfLastScan = millis(); // One way to check how attemptTransmission worked out if (tcpIpNode.latestTransmissionSuccessful()) { Serial.println(F("Transmission successful.")); } // Another way to check how attemptTransmission worked out if (tcpIpNode.latestTransmissionOutcomes().empty()) { Serial.println(F("No mesh AP found.")); } else { for (TransmissionOutcome &transmissionOutcome : tcpIpNode.latestTransmissionOutcomes()) { if (transmissionOutcome.transmissionStatus() == TransmissionStatusType::TRANSMISSION_FAILED) { Serial.println(String(F("Transmission failed to mesh AP ")) + transmissionOutcome.SSID()); } else if (transmissionOutcome.transmissionStatus() == TransmissionStatusType::CONNECTION_FAILED) { Serial.println(String(F("Connection failed to mesh AP ")) + transmissionOutcome.SSID()); } else if (transmissionOutcome.transmissionStatus() == TransmissionStatusType::TRANSMISSION_COMPLETE) { // No need to do anything, transmission was successful. } else { Serial.println(String(F("Invalid transmission status for ")) + transmissionOutcome.SSID() + String('!')); assert(F("Invalid transmission status returned from responseHandler!") && false); } } } Serial.println(); } else { /* Accept any incoming connections */ tcpIpNode.acceptRequests(); } }
#include <ESP8266WiFi.h> #include <DNSServer.h> #include <ESP8266WebServer.h> #include <WiFiManager.h> #include <Ticker.h> // Instancia a la clase Ticker Ticker ticker; // Pin LED azul byte pinLed = D4; void parpadeoLed(){ // Cambiar de estado el LED byte estado = digitalRead(pinLed); digitalWrite(pinLed, !estado); } void setup() { Serial.begin(115200); // Modo del pin pinMode(pinLed, OUTPUT); // Empezamos el temporizador que hará parpadear el LED ticker.attach(0.2, parpadeoLed); // Creamos una instancia de la clase WiFiManager WiFiManager wifiManager; // Descomentar para resetear configuración //wifiManager.resetSettings(); // Cremos AP y portal cautivo y comprobamos si // se establece la conexión if(!wifiManager.autoConnect("ESP8266Temp")){ Serial.println("Fallo en la conexión (timeout)"); ESP.reset(); delay(1000); } Serial.println("Ya estás conectado"); // Eliminamos el temporizador ticker.detach(); // Apagamos el LED digitalWrite(pinLed, HIGH); } void loop() {}
WifiManager
#include <ESP8266WiFi.h> //https://github.com/esp8266/Arduino //needed for library #include <DNSServer.h> #include <ESP8266WebServer.h> #include "WiFiManager.h" //https://github.com/tzapu/WiFiManager std::unique_ptr<ESP8266WebServer> server; void handleRoot() { server->send(200, "text/plain", "hello from esp8266!"); } void handleNotFound() { String message = "File Not Found\n\n"; message += "URI: "; message += server->uri(); message += "\nMethod: "; message += (server->method() == HTTP_GET) ? "GET" : "POST"; message += "\nArguments: "; message += server->args(); message += "\n"; for (uint8_t i = 0; i < server->args(); i++) { message += " " + server->argName(i) + ": " + server->arg(i) + "\n"; } server->send(404, "text/plain", message); } void setup() { // put your setup code here, to run once: Serial.begin(115200); //Serial.setDebugOutput(true); //WiFiManager //Local intialization. Once its business is done, there is no need to keep it around WiFiManager wifiManager; //reset saved settings wifiManager.resetSettings(); //fetches ssid and pass from eeprom and tries to connect //if it does not connect it starts an access point with the specified name //here "AutoConnectAP" //and goes into a blocking loop awaiting configuration //wifiManager.autoConnect("AutoConnectAP"); //or use this for auto generated name ESP + ChipID wifiManager.autoConnect(); //if you get here you have connected to the WiFi Serial.println("connected...yeey :)"); server.reset(new ESP8266WebServer(WiFi.localIP(), 80)); server->on("/", handleRoot); server->on("/inline", []() { server->send(200, "text/plain", "this works as well"); }); server->onNotFound(handleNotFound); server->begin(); Serial.println("HTTP server started"); Serial.println(WiFi.localIP()); } void loop() { // put your main code here, to run repeatedly: server->handleClient(); }